A Beginner’s Guide to Commonly Mixed-Up JavaScript Symbols
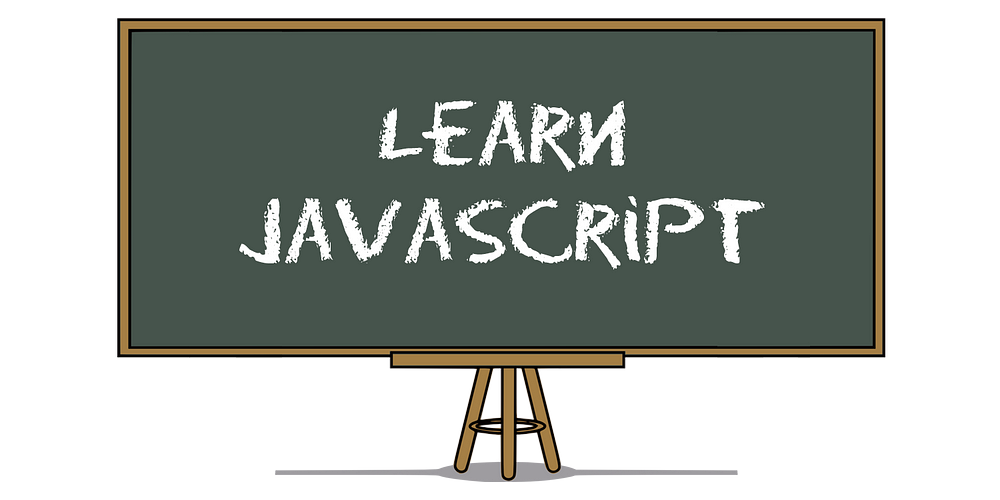
Having taught programming and working with business users who are not daily coders, it can be challenging to remember “which is which” when it comes to different JavaScript symbols. I’ve outlined three sets of commonly confused symbols to ease the learning curve.
Before we jump in, I want to define a few programming terms for the uninitiated. If these terms are new, it’s worth the time to learn them because you’ll see them time and time again. Plus, knowing the definition will help you conceptually understand what’s going on as well.
- Operator: An operator is a character or set of characters that perform an action. The simplest example is the addition operator (+) which will add two numeric values together. The important takeaway here is that an operator does something. Think of an operator as a verb instead of a comma or other structural punctuation.
- Boolean Expression: A boolean expression, whch I’ll refer to simply as an expression, is a piece of code that evaluates to true or false. For example, if I say “today is Tuesday” that is either correct or incorrect. Boolean expressions can be very simple and exceedingly complex when you combine multiple simple expressions. For now let’s keep things simple and think of a boolean expression as an operator with two operands (values that are operated on) such as
x < 7
. - Function: A function is a defined set of instructions that has a consistent input and output. Think of a function as a black box where all the code needs to know is what to put in and what to expect out. A function’s definition is the code where the function’s steps are written out. A function call is where you invoke the function’s definition to be executed. Simple analogy: create a plan = function definition; execute the plan = function call.
Okay, now that we have those terms defined, let’s get to the three pairs of commonly confused JavaScript symbols!
= vs ==
The equal sign and double equal sign are two operators that you could easily mistake at a quick glance but serve very different purposes.
Let’s start by giving each a name. The single equal sign is called the assignment operator. Its purpose is to take the value on the right side and store it in the left. The double equal sign is called the equality operator. Its purpose is to check if the value on the right is equal to the value on the left.
BONUS: The triple equal sign is called a strict equality operator and is a more restrictive version of the double equal sign. We haven’t talked about data types and they are central to the strict equality operator because in addition to the value, the data types must match for a true evaluation. The easiest example is "1" == 1
vs "1" === 1
. The first example would return true whereas the second would evaluate false. Check out Brandon Morelli’s article for more information.
|| vs &&
These two sets of symbols are called logical operators. They don’t get visually confused but rather it’s challenging to remember which to use. Their purpose is to connect two expressions and evaluate the overall truth.
The double vertical bar, also called a pipe, is the logical OR operator. Its purpose is to evaluate both expressions where only one must be true. The double ampersand is the logical AND operator. Its purpose is to evaluate both expressions where both must be true.
Let’s use the date Monday, April 1, 2019 to explain the difference between the two. I’m going to write a few sentences and your job is to spot the lie.
- It is Monday or Tuesday
- It is Monday and June
- It is June or July
- It is a weekday and 2019
The sentences where I told a lie (2 and 3) would evaluate to false. The truthful sentences (1 and 4) would evaluate to true.
Why is this important? Using ||
and &&
allow you to join simple expressions into compound expressions that express more complicated logic. If you need a number with a certain range, say from 1–10, you can validate with a compound expression:
x >= 1 && x <= 10
What if you use the wrong logical operator? If you used the double pipe then only one side of the expression has to be valid for the overall expression to be true. So if x was -1, it fails the first expression; however, -1 is less than or equal to 10 so the overall expression evaluates as true.
BONUS: You can have a three-part compound expressions such as x == 1 || x == 2 || x == 3
as long as a single expression is true in a series of logical OR operators, the overall evaluation is true. Similarly, in a series of logical AND operators, only one false expression will cause the overall evaluation to be false.
( ) vs { }
Parentheses and curly braces (also called curly brackets) don’t have fancy operator names, but that’s because they don’t actually perform any sort of operation. Both sets of characters are used to enclose other content. The key is knowing when to use each one.
Parentheses have two primary functions. The first is just like your grade school arithmetic classes where parentheses take priority in the order of operations. So if we wanted to store the result of 5 + 4 multiplied by 2 in the variable x, it would look like:x = (5 + 4) * 2
in case you’re rusty, without the parenthesis 4 and 2 would be multiplied first then added to 5.
The second use of parentheses is to include additional information to a command such as an expression to an if statement or variables to a function. I’ll focus on function definitions because the former will be quite clear as we work through it. When defining a function, it will often require inputs — technically named parameters. For example, to calculate the area of a rectangle you need to know the length and the width… those would be parameters. This is what a function definition looks like:
function calculateArea(length,width) {
var area = length * width;
return area;
}
Similarly, when you call or use the function, you must pass values that will be stored in the parameters. These values you pass are called arguments. Using the function might look like this:
bedroom = calculateArea(12,15)
livingroom = calculateArea(20,8)
What if the function does not have any parameters and thus requires no arguments? Then you have empty parentheses:
function printWelcome() {
print("Welcome to the show!");
}printWelcome()
Now I know I jumped the gun a bit because I used curly braces in the parentheses example… If you didn’t realize, the curly braces are used to define what are called code blocks. In the above examples the curly braces define they start and end of the function’s definition. Curly braces are also used to define the start and end of if statements, if/else statements, and while loops to name a few.
BONUS: If you see an if statement without curly braces it isn’t necessarily wrong. Languages are continually improving ease-of-use and sometimes that means allowing shortcuts. If the statements are in the same line as the if definition then curly braces are not required.
if(month == "January") print("It's your birthday month");
Now it’s time to put it all together!
function partyTime() {
print("It's Party Time!");
}today = "Friday";if(today == "Friday" || today == "Saturday") {
partyTime();
}
Hopefully you were able to pick out the following:
- assignment operator to set today equal to Friday
- equality operator to compare the value of today
- logical OR operator to connect two simple expressions
- parentheses when defining partyTime
- parentheses to call the print function and partyTime function
- parentheses to include the expression for the if statement
- curly braces to define the code block when the if statement evaluates true
This is really just the tip of the iceberg, but when you don’t regularly live in code it can be hard to look at a snippet and easily read it. Please leave questions below in the comments. Please clap and/or share if you found the article helpful.